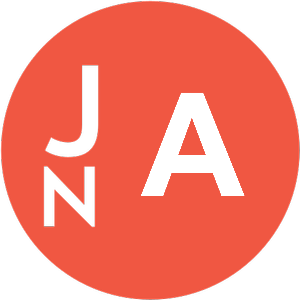
This a simple authentication implementation for ASP.NET Core. It was implemented in order to facilitate the use of the simplest and most common authentication methods in ASP.NET core applications. Today it is in use in some web API projects.
The supported authentication schemes are:
- Basic Authentication Scheme
- API Key Custom Authentication Scheme
Current version
Current version is 1.3.0
Release notes for current version
- added ContentType property to ChallengeResult object returned by delegate ChallengeResponse. This is useful to change response content type to “application/problem+json” if the content is a ProblemDetails object.
- added information about the HTTP request to ChallengeResponse delegate (RequestDetails object)
- updated dependencies
Install
Download the package from NuGet:
Install-Package JN.Authentication -version [version number]
The package is available here and source code is available here.
Usage
First, you must add one (or both) authentication scheme to the application pipeline:
public void ConfigureServices(IServiceCollection services)
{
// Basic authentication
services.AddAuthentication(BasicAuthenticationDefaults.AuthenticationScheme)
.AddBasic(options =>
{
options.Realm = "api";
options.LogInformation = true; //optional, default is false;
options.HttpPostMethodOnly = false;
options.HeaderEncoding = Encoding.UTF8; //optional, default is UTF8;
options.ChallengeResponse = ValidationService.ChallengeResponse;
});
// validation service
services.AddSingleton<IBasicValidationService, BasicValidationService>();
// ApiKey authentication
services.AddAuthentication(ApiKeyAuthenticationDefaults.AuthenticationScheme)
.AddApiKey(options =>
{
options.LogInformation = true;
options.HttpPostMethodOnly = false;
options.AcceptsQueryString = true;
options.HeaderName = "ApiKey";
options.ChallengeResponse = ValidationService.ChallengeResponse;
});
// validation service
services.AddSingleton<IApiKeyValidationService, ApiKeyValidationService>();
}
ChallengeResponse
is a delegate called before a 401 response is sent to the client. It can be used to change the response (including content type). You can see an example here.
IBasicValidationService
and IApiKeyValidationService
should have an implementation where the access details are validated (for example by querying a database).
An implementation for ValidateUser
of interface IBasicValidationService
could be something like the following:
public async Task<ValidationResult> ValidateUser(string username, string password, string resourceName)
{
var user = await GetFromDB(username, password, resourceName);
if (user != null)
{
var claims = new[]
{
new Claim(ClaimTypes.GivenName, user.FullName),
new Claim(ClaimTypes.Name, username),
new Claim(ClaimTypes.Email, user.Email),
new Claim("IsAdmin", user.IsAdmin),
new Claim(ClaimTypes.Role, user.Roles)
};
var res = new ValidationResult
{
Success = true,
Claims = claims
};
return res;
}
return new ValidationResult
{
Success = false,
ErrorDescription = "Invalid User",
ErrorCode = -1
};
}
On your controllers add the Authorize
atribute and choose the Authentication Scheme (“Basic” or “ApiKey”).
[Route("api/[controller]")]
[Authorize(AuthenticationSchemes = "Basic", Policy = "IsAdminPolicy")]
[ApiController]
public class BasicAuthSchemeTestController : ControllerBase
{
// Your code here
}
Options
Both authentication schemes allows to:
LogInformation
: log information using a logging providerHttpPostMethodOnly
: allows only POST requests
Basic allows to specify a Realm
and HeaderEncoding
.
ApiKey authentication allows to change the HeaderName
(default is “ApiKey”) and can also accept the key in the query string (AcceptsQueryString
)